Using Servo Motor with ESP32
In this article, we will see how to connect the servo motor to ESP32 and how to program it.
Servo motors are actually able to rotate to particular angle and so that we can create a ton of projects with it. On the other hand, there are some servo motors that we can run on specific speed, and direction. But before we dive into the projects, we will see some basics first.
Just to mention it, I'm using a "continuous rotation servo", so I can manage the speed and direction of it, but not the angle (not directly at least).
The other type (that I am not using currently) is called "standard positional servo", which can run and turn on specific angles. But you cannot manage the speed of the rotation.
Wiring up the ESP32
Connect everything as below
For me, the brown wire is ground, so I'll connect it with ground pin of ESP32.
The middle wire (red, in my case!) is for voltage, and I'll connect it to VIN
pin, which is 5V. I've a small servo motor, which is also fine with 3.5V, but I'll connect it with 5V anyway.
The last one (yellow wire) will be connected to one of the GPIO pins, in my case I'm connecting it to D25
.
Coding
First, open the Arduino IDE, we will install a library for the servo motor.
Installing the library
Go to Tools
>Manage Libraries...
and then search ESP32 Servo Kevin
and download the one shown below.
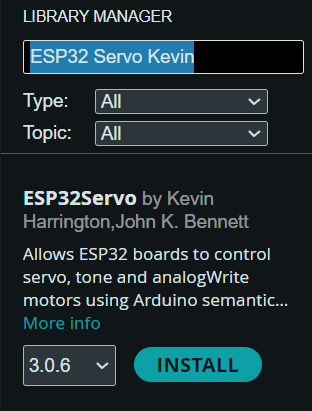
Source code
#include <ESP32Servo.h>
Servo myServo;
const int servoPin = 25;
void setup() {
myServo.attach(servoPin, 1000, 2000); // Adjust min/max PWM for continuous servo
myServo.write(90); // Stop the servo initially
delay(1000);
}
void loop() {
// Rotate clockwise for 1 second
myServo.write(180);
delay(1000);
// stop for 1 second
myServo.write(90);
delay(1000);
// Rotate anti-clockwise for 2 seconds
myServo.write(0);
delay(2000);
// stop for 1 second
myServo.write(90);
delay(1000);
}
Results
Thank you very much for reading this article, see you in the next one.